Tutorial¶
Getting started¶
To start using viznet, simply
$ pip install viznet
or clone/download this repository and run
$ cd viznet/
$ pip install -r requirements.txt
$ python setup.py install
Node and Edge Brush¶
viznet focuses on node-edge based graphs. Instead of directly drawing nodes and edges, a brush system is used. The following code examplify how to draw two nodes and connection them using a directed edge.
>> from viznet import NodeBrush, EdgeBrush, DynamicShow
>> with DynamicShow() as d:
>> brush = NodeBrush('nn.input', size='normal')
> node1 = brush >> (1,0) # paint a node at (x=1, y=0)
>> node2 = brush >> (2,0)
>> edge = EdgeBrush('->-', lw=2)
>> edge >> (node1, node2) # connect two nodes
>> node1.text('First', 'center', fontsize=18) # add text to node1
>> node2.text('Second', 'center', fontsize=18)
DynamicShow
is a utility class that automatically equalize axes and then remove axes to make graph clean. NodeBrush
take the style string as its first argument, besides elementary styles like basic and invisible styles, styles for neural network (nn.) and tensor network (tn.) are defined as
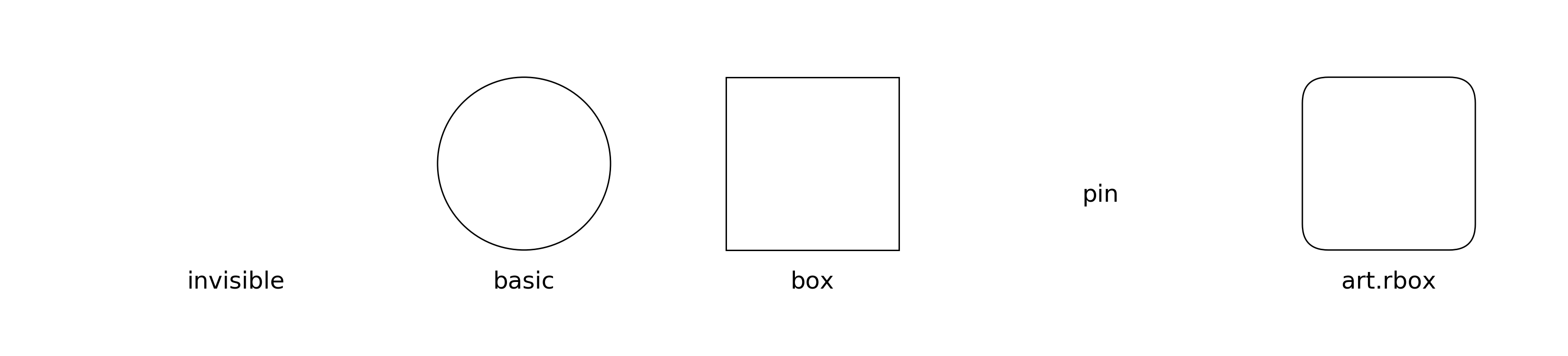
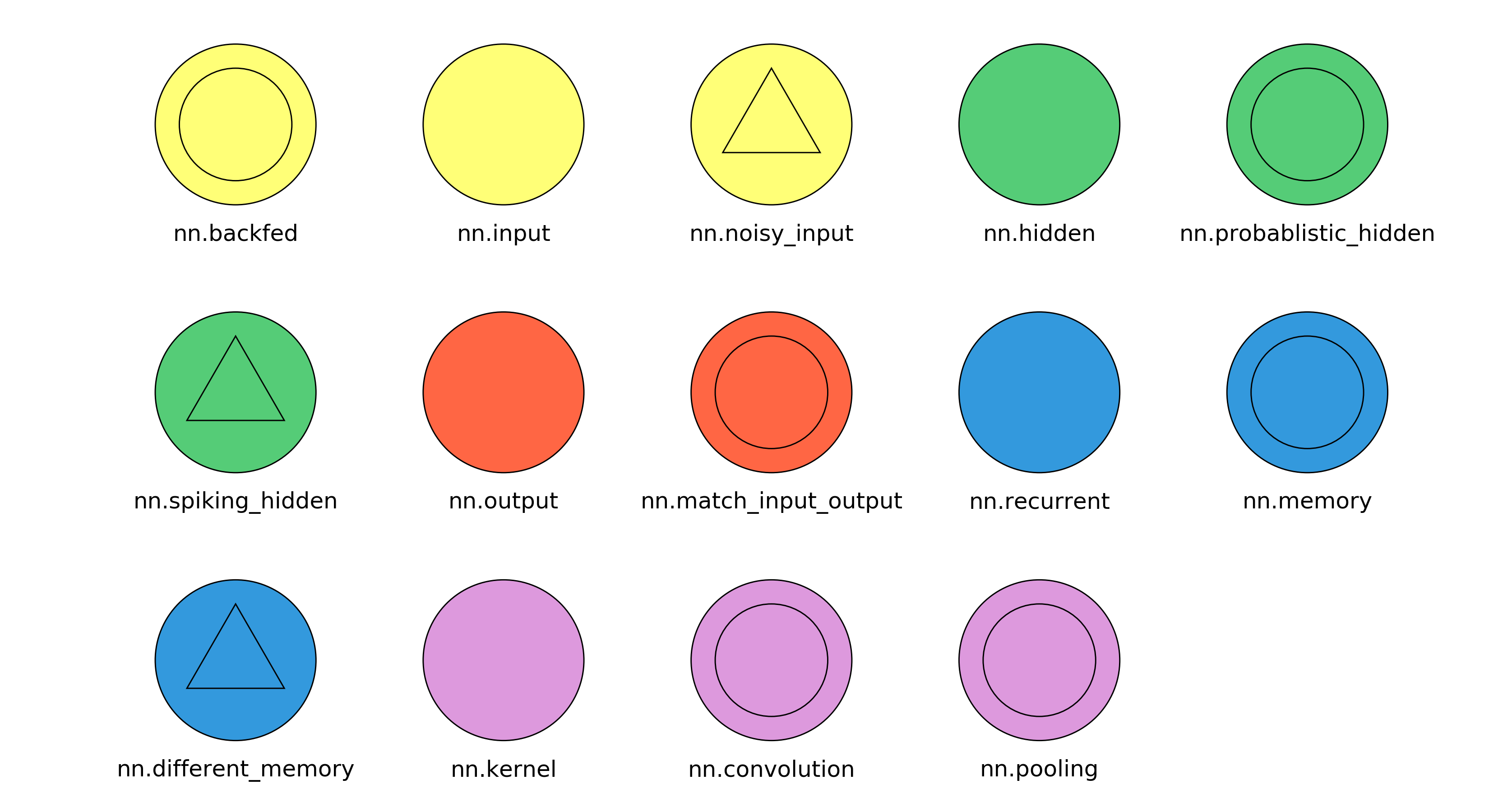
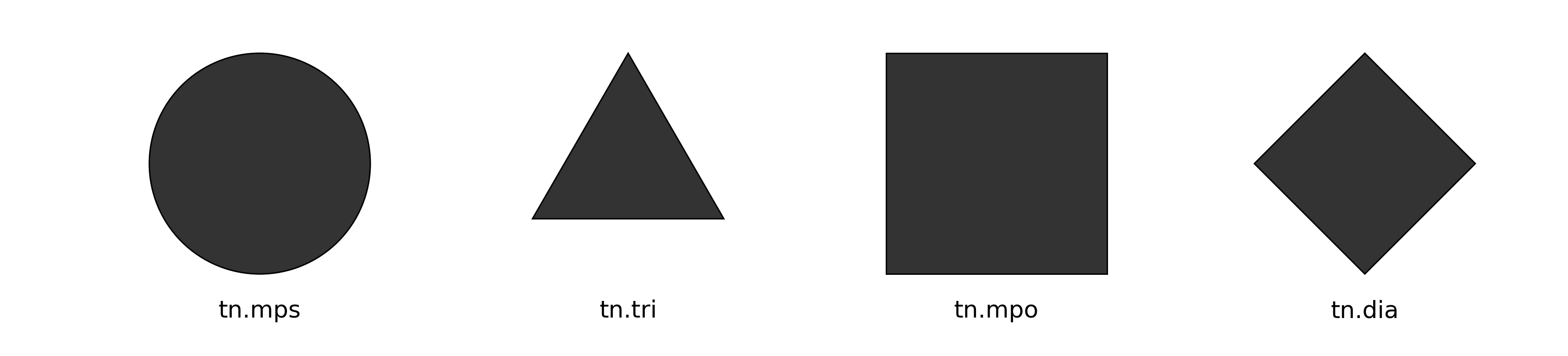
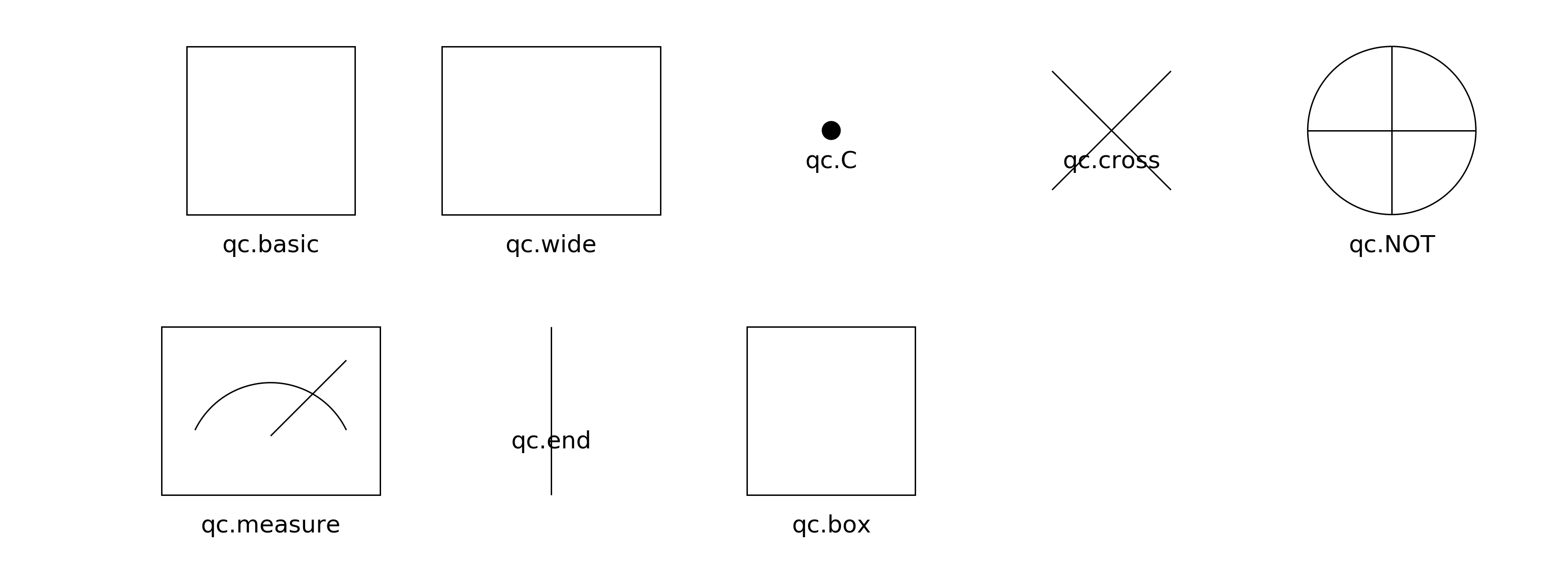
EdgeBrush
(orCLinkBrush
, the curvy edge class) take a string as style, this must must be composed of characters in [ - | . | = | > | < ],- ‘-‘: solid line,
- ‘=’: double solid line,
- ‘.’: dashed line,
- ‘>’: arrow towards end of line, no length,
- ‘<’: arrow towards start of line, no length.
For example,
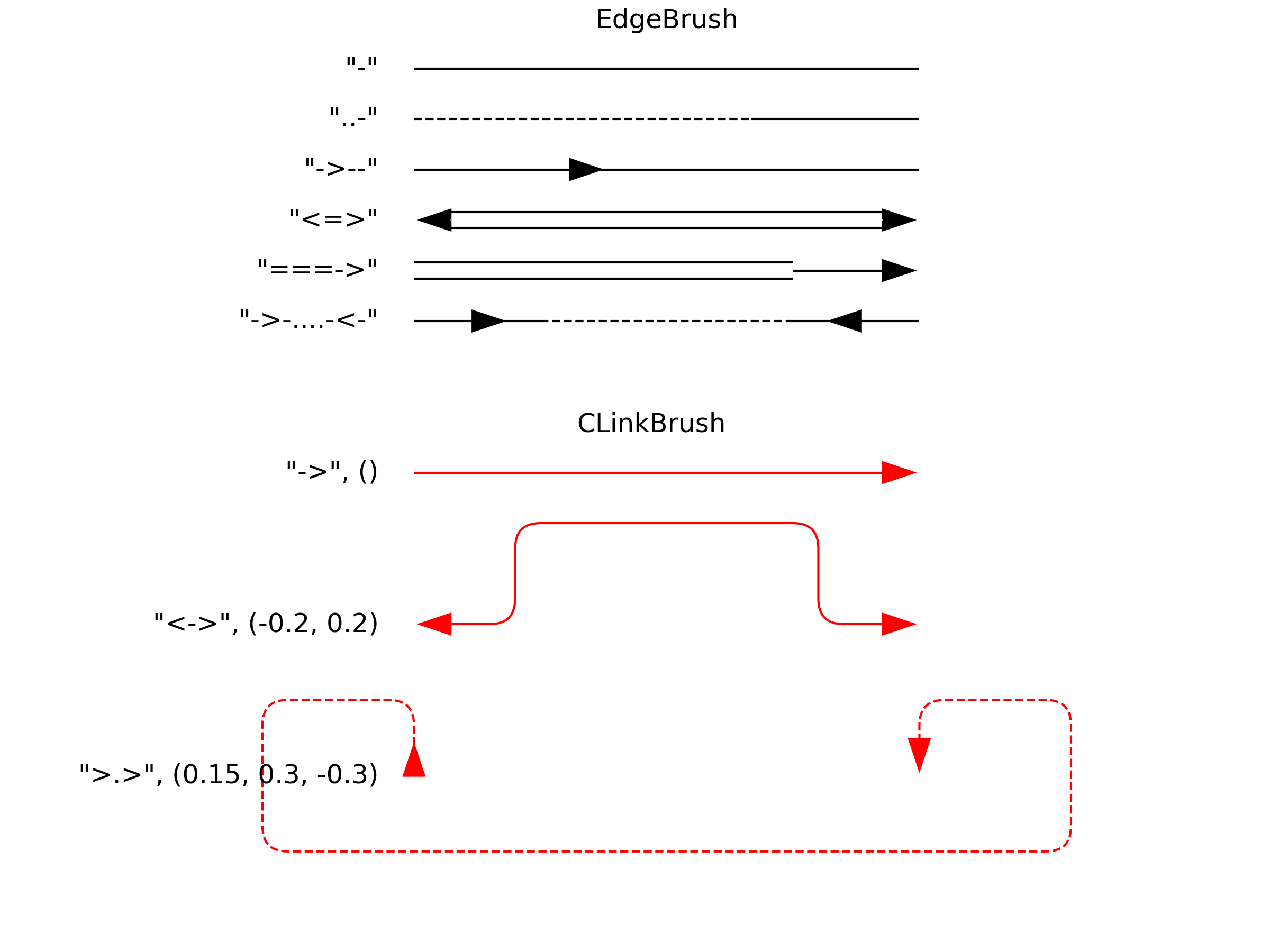
In this example, the CLinkBrush
instances use the roundness of 0.2 (default is 0) to round the turning points, while the grow directions of lines are controled by offsets.
Also, you can set color and width of your line for this EdgeBrush
by passing arguments into construction method.
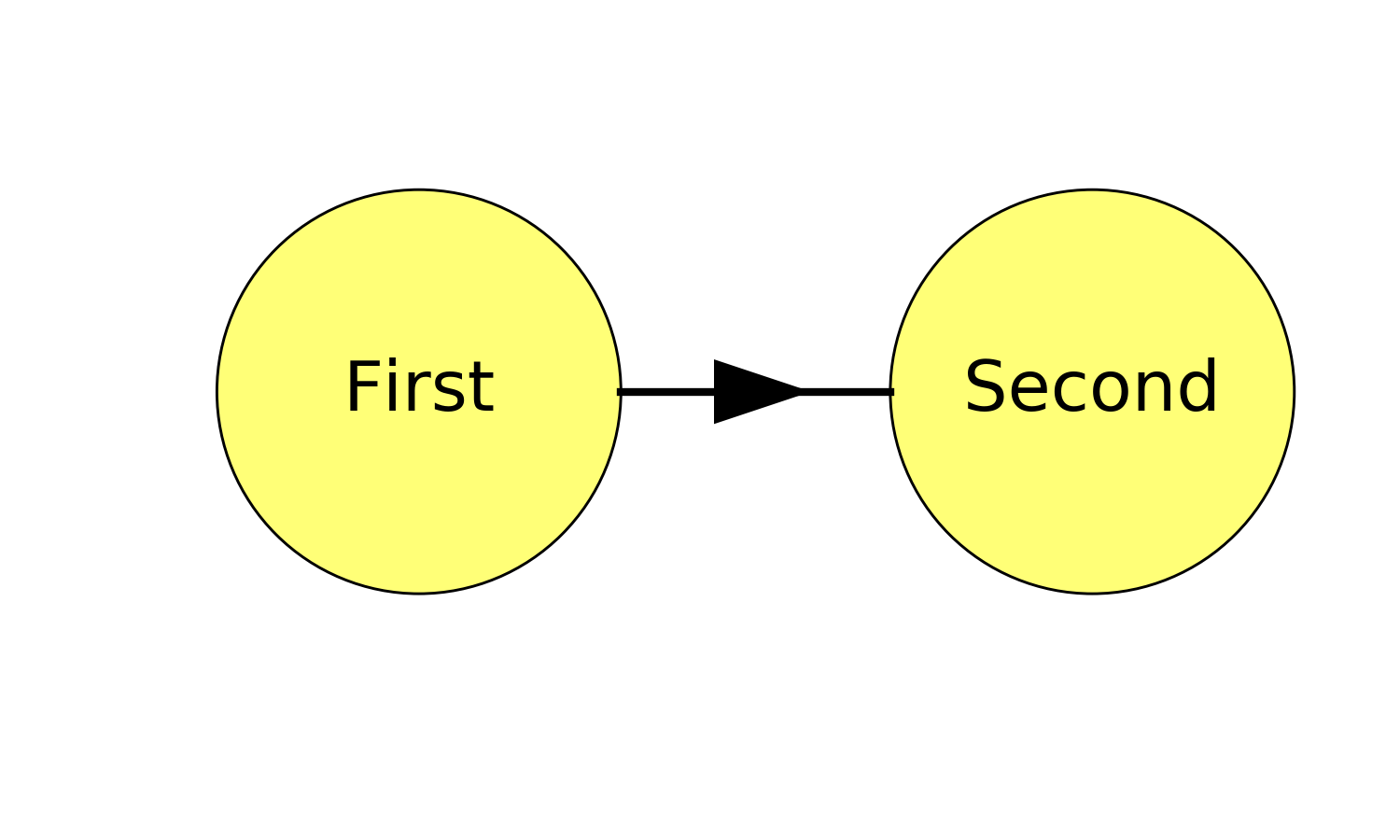
Pins¶
The above naive connection may not be what you want, pinning is needed. A pin is a special node, with no size, and is designed for connecting edges. Let’s continue the above example,
>> mpo21 = NodeBrush('tn.mpo', size='normal')
>> mpo21.size = (0.7, 0.3)
>> node3 = mpo21 >> (1.5, 1.0)
>> left_bottom_pin = node3.pin('bottom', align=node1)
>> edge >> (left_bottom_pin, node1)
Now, your canvas looks like
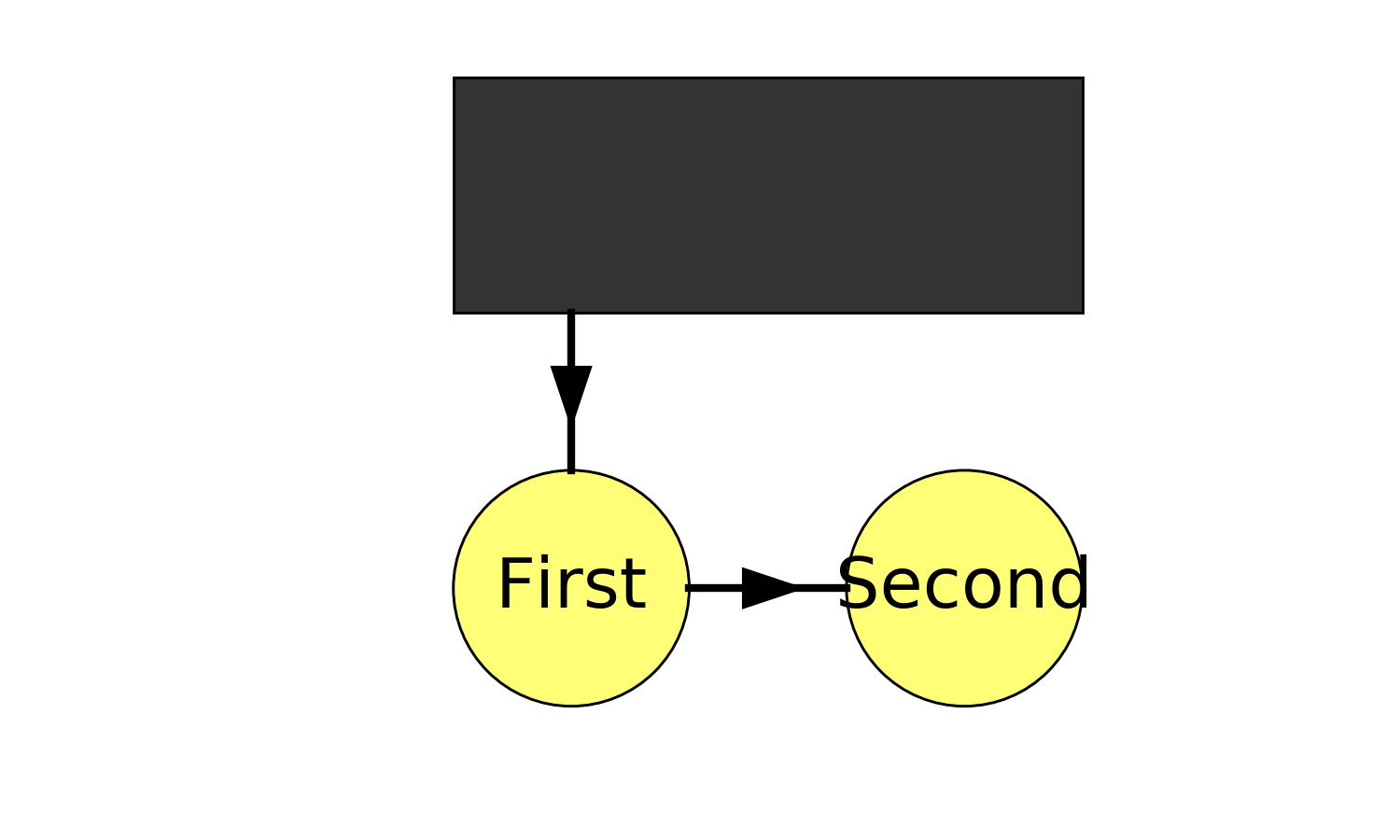
More¶
Cluster operations like one to one connections and all to all connections between different layers in neural network are frequently used APIs.
For a quantum circuit, we also have a facility viznet.QuantumCircuit
to help us build it easily.
To learn more, you may go through this notebook
.
Read some examples under path/to/viznet/apps/nn/ and path/to/viznet/apps/qc/ to learn about them. Also, Examples chapter of this documentation gives some examples.